Introduction In this article, we will explore how to create a simple slot machine game using JavaScript. This project combines basic HTML structure for layout, CSS for visual appearance, and JavaScript for the logic of the game. Game Overview The slot machine game is a classic casino game where players bet on a set of reels spinning and displaying symbols. In this simplified version, we will use a 3x3 grid to represent the reels, with each cell containing a symbol (e.g., fruit, number). The goal is to create a winning combination by matching specific sets of symbols according to predefined rules.
Beste casinoer india 2024
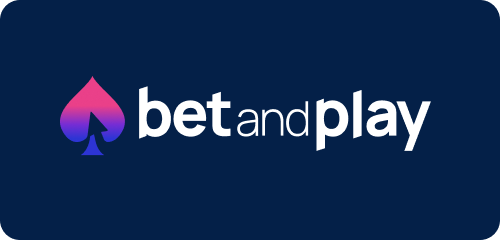
- 24/7 live chat
- Spesielt VIP-program
- Royal Wins
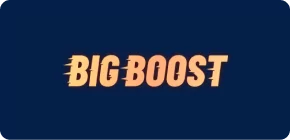
- Regular promotions
- Deposit with Visa
- Luck&Luxury
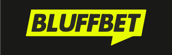
- Regular promotions
- Deposit with Visa
- Celestial Bet
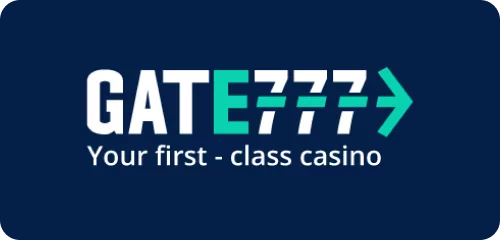
- Regular promotions
- Deposit with Visa
- Win Big Now
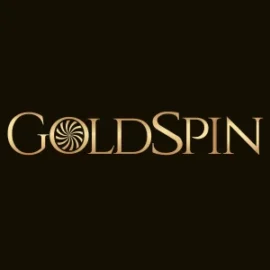
- Regular promotions
- Deposit with Visa
- Elegance+Fun
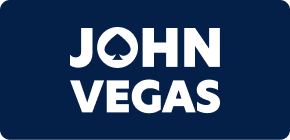
- Regular promotions
- Deposit with Visa
- Luxury Play
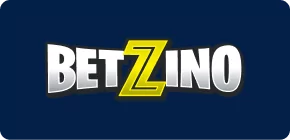
- Regular promotions
- Deposit with Visa
- Opulence & Thrills
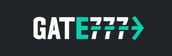
- Regular promotions
- Deposit with Visa
- Luck&Luxury
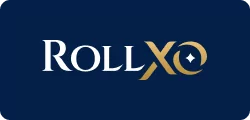
- Regular promotions
- Deposit with Visa
- Opulence & Fun
- create a javascript slot machine
- slot machine powerpoint template
- keno cards printable free
- create custom scratch offs: diy scratch off maker guide
- About create a javascript slot machine FAQ
create a javascript slot machine
Introduction
In this article, we will explore how to create a simple slot machine game using JavaScript. This project combines basic HTML structure for layout, CSS for visual appearance, and JavaScript for the logic of the game.
Game Overview
The slot machine game is a classic casino game where players bet on a set of reels spinning and displaying symbols. In this simplified version, we will use a 3x3 grid to represent the reels, with each cell containing a symbol (e.g., fruit, number). The goal is to create a winning combination by matching specific sets of symbols according to predefined rules.
Setting Up the HTML Structure
Firstly, let’s set up the basic HTML structure for our slot machine game. We will use a grid container (<div>
) with three rows and three columns to represent the reels.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>JavaScript Slot Machine</title>
<link rel="stylesheet" href="styles.css">
</head>
<body>
<!-- Game Container -->
<div id="game-container">
<!-- Reels Grid -->
<div class="reels-grid">
<!-- Reel 1 Row 1 -->
<div class="reel-cell symbol-1"></div>
<div class="reel-cell symbol-2"></div>
<div class="reel-cell symbol-3"></div>
<!-- Reel 2 Row 1 -->
<div class="reel-cell symbol-4"></div>
<div class="reel-cell symbol-5"></div>
<div class="reel-cell symbol-6"></div>
<!-- Reel 3 Row 1 -->
<div class="reel-cell symbol-7"></div>
<div class="reel-cell symbol-8"></div>
<div class="reel-cell symbol-9"></div>
<!-- Reel 1 Row 2 -->
<div class="reel-cell symbol-10"></div>
<div class="reel-cell symbol-11"></div>
<div class="reel-cell symbol-12"></div>
<!-- Reel 2 Row 2 -->
<div class="reel-cell symbol-13"></div>
<div class="reel-cell symbol-14"></div>
<div class="reel-cell symbol-15"></div>
<!-- Reel 3 Row 2 -->
<div class="reel-cell symbol-16"></div>
<div class="reel-cell symbol-17"></div>
<div class="reel-cell symbol-18"></div>
<!-- Reel 1 Row 3 -->
<div class="reel-cell symbol-19"></div>
<div class="reel-cell symbol-20"></div>
<div class="reel-cell symbol-21"></div>
<!-- Reel 2 Row 3 -->
<div class="reel-cell symbol-22"></div>
<div class="reel-cell symbol-23"></div>
<div class="reel-cell symbol-24"></div>
<!-- Reel 3 Row 3 -->
<div class="reel-cell symbol-25"></div>
<div class="reel-cell symbol-26"></div>
<div class="reel-cell symbol-27"></div>
</div>
</div>
<script src="script.js"></script>
</body>
</html>
Setting Up the CSS Style
Next, we will set up the basic CSS styles for our slot machine game.
/* Reels Grid Styles */
.reels-grid {
display: grid;
grid-template-columns: repeat(3, 1fr);
grid-gap: 10px;
}
/* Reel Cell Styles */
.reel-cell {
height: 100px;
width: 100px;
border-radius: 20px;
background-color: #333;
display: flex;
justify-content: center;
align-items: center;
}
.symbol-1, .symbol-2, .symbol-3 {
background-image: url('img/slot-machine/symbol-1.png');
}
.symbol-4, .symbol-5, .symbol-6 {
background-image: url('img/slot-machine/symbol-4.png');
}
/* Winning Line Styles */
.winning-line {
position: absolute;
top: 0;
left: 0;
width: 100%;
height: 2px;
background-color: #f00;
}
Creating the JavaScript Logic
Now, let’s create the basic logic for our slot machine game using JavaScript.
// Get all reel cells
const reelCells = document.querySelectorAll('.reel-cell');
// Define symbols array
const symbolsArray = [
{ id: 'symbol-1', value: 'cherry' },
{ id: 'symbol-2', value: 'lemon' },
{ id: 'symbol-3', value: 'orange' },
// ...
];
// Function to spin the reels
function spinReels() {
const winningLine = document.querySelector('.winning-line');
winningLine.style.display = 'none';
reelCells.forEach((cell) => {
cell.classList.remove('symbol-1');
cell.classList.remove('symbol-2');
// ...
const newSymbol = symbolsArray[Math.floor(Math.random() * 27)];
cell.classList.add(newSymbol.id);
// ...
});
}
// Function to check winning combinations
function checkWinningCombinations() {
const winningLine = document.querySelector('.winning-line');
const symbolValues = reelCells.map((cell) => cell.classList.value.split(' ')[1]);
if (symbolValues.includes('cherry') && symbolValues.includes('lemon') && symbolValues.includes('orange')) {
winningLine.style.display = 'block';
// Add win logic here
}
}
// Event listener to spin the reels
document.getElementById('spin-button').addEventListener('click', () => {
spinReels();
checkWinningCombinations();
});
Note: The above code snippet is for illustration purposes only and may not be functional as is.
This article provides a comprehensive guide on creating a JavaScript slot machine game. It covers the basic HTML structure, CSS styles, and JavaScript logic required to create this type of game. However, please note that actual implementation might require additional details or modifications based on specific requirements or constraints.
slot machine powerpoint template
Creating a captivating and professional presentation can be a daunting task, especially when you’re dealing with topics related to online entertainment, gambling, or casinos. A well-designed PowerPoint template can make all the difference in engaging your audience and effectively conveying your message. If your presentation revolves around slot machines, electronic gaming, or the casino industry, a Slot Machine PowerPoint Template could be the perfect choice.
Why Choose a Slot Machine PowerPoint Template?
A Slot Machine PowerPoint Template offers several advantages:
- Theme Relevance: It aligns perfectly with topics related to gambling, casinos, and online entertainment.
- Visual Appeal: The design elements can make your presentation more engaging and memorable.
- Professionalism: A well-crafted template can enhance the overall professionalism of your presentation.
- Time-Saving: Pre-designed layouts and graphics save you time and effort in creating slides from scratch.
Key Features of a Slot Machine PowerPoint Template
When selecting a Slot Machine PowerPoint Template, look for the following features:
1. Design Elements
- Slot Machine Graphics: High-quality images or vector graphics of slot machines.
- Casino Icons: Icons representing coins, cards, dice, and other casino-related symbols.
- Color Scheme: A vibrant color palette that reflects the excitement of gambling.
2. Slide Layouts
- Title Slides: Eye-catching designs for the opening slide.
- Content Slides: Various layouts for text, images, and charts.
- Transition Slides: Special slides for transitions or section breaks.
3. Customization Options
- Editable Text: Easily modify text to fit your content.
- Resizable Graphics: Adjust the size and position of images and icons.
- Color Customization: Change the color scheme to match your branding or preference.
How to Use a Slot Machine PowerPoint Template
1. Download the Template
- Choose a reliable source to download the template. Websites like Envato Elements, SlideModel, or GraphicRiver offer high-quality PowerPoint templates.
2. Open in PowerPoint
- Once downloaded, open the template in PowerPoint.
- Familiarize yourself with the different slide layouts available.
3. Customize the Content
- Replace placeholder text with your own content.
- Insert your images, charts, and other visual elements.
- Customize the color scheme if necessary.
4. Review and Finalize
- Review all slides to ensure consistency and clarity.
- Make any final adjustments to text, images, or layout.
- Save your presentation in the desired format (e.g., PPTX, PDF).
Best Practices for Using a Slot Machine PowerPoint Template
1. Keep It Simple
- Avoid overloading slides with too much text or graphics.
- Use bullet points for clarity and readability.
2. Maintain Consistency
- Ensure that all slides follow the same design theme.
- Use consistent fonts and colors throughout the presentation.
3. Engage Your Audience
- Use animations sparingly to maintain focus.
- Incorporate interactive elements if appropriate.
4. Practice Your Presentation
- Rehearse your presentation to ensure smooth delivery.
- Time your slides to match your speaking pace.
A Slot Machine PowerPoint Template can significantly enhance your presentation on topics related to gambling, casinos, and online entertainment. By choosing a template with relevant design elements, customizable options, and professional layouts, you can create an engaging and memorable presentation. Remember to keep your content clear, consistent, and visually appealing to effectively communicate your message to your audience.

keno cards printable free
Keno is a popular lottery-style game that has been enjoyed by millions of people worldwide. Whether you’re playing at a casino or hosting a home game, having printable Keno cards can make the experience more enjoyable and organized. In this article, we’ll explore where you can find free printable Keno cards, how to use them, and some tips to enhance your Keno gameplay.
Where to Find Free Printable Keno Cards
Finding free printable Keno cards is easier than you might think. Here are some reliable sources:
Online Casino Websites: Many online casinos offer free printable Keno cards as part of their promotional materials. These cards are usually high-quality and designed to mimic the experience of playing at a real casino.
Gaming Forums and Communities: Websites like Reddit and specialized gaming forums often have members sharing free printable Keno cards. These can be a great resource, especially if you’re looking for unique designs.
Printable Template Websites: Websites such as Template.net and PrintablePaper.net offer a variety of free printable templates, including Keno cards. These sites often have user-friendly interfaces and high-quality designs.
Google Search: A simple Google search for “free printable Keno cards” can yield numerous results. Be sure to check the credibility of the website before downloading any files.
How to Use Printable Keno Cards
Using printable Keno cards is straightforward and can add a touch of authenticity to your Keno game. Here’s how to do it:
Download the Template: Choose a Keno card template from one of the sources mentioned above. Download the file to your computer.
Print the Cards: Use a high-quality printer to print the cards. Ensure that your printer settings are set to the highest quality for the best results.
Prepare Your Game: Before starting the game, decide on the number of players and the rules you’ll be using. Each player should have their own Keno card.
Mark Your Numbers: As the numbers are drawn, players mark their cards with a pen or pencil. Some players prefer using bingo daubers for a more authentic casino feel.
Check for Wins: After the drawing, compare your marked numbers to the drawn numbers. If you have a winning combination, you’ve won!
Tips for Enhancing Your Keno Gameplay
To make your Keno game more enjoyable and strategic, consider these tips:
Set a Budget: Before starting, decide on a budget for your game. This will help you manage your spending and ensure that everyone has a good time.
Use Multiple Cards: Some players find it more exciting to use multiple Keno cards. This increases your chances of winning but also requires more attention.
Track Your Numbers: Keep a record of the numbers that have been drawn. This can help you identify patterns and make more informed choices in future games.
Host a Themed Event: Consider hosting a Keno night with a specific theme. This can add an extra layer of fun and make the event more memorable.
Printable Keno cards are a fantastic way to bring the excitement of the casino to your home. With the right resources and a bit of preparation, you can create a fun and engaging Keno experience for you and your friends. Whether you’re a seasoned player or new to the game, printable Keno cards can enhance your gameplay and make every session more enjoyable.
create custom scratch offs: diy scratch off maker guide
Creating custom scratch offs is a fun and engaging way to add excitement to various events, promotions, or personal projects. Whether you’re organizing a casino night, planning a marketing campaign, or just looking for a unique gift idea, this DIY scratch off maker guide will help you bring your vision to life.
Materials Needed
Before you start, gather the following materials:
- Scratch Off Coating: This is the key ingredient for your scratch offs. You can find scratch off coating in craft stores or online.
- Inkjet Printer: For printing your designs onto the scratch off material.
- Cardstock or Heavy Paper: The base material for your scratch offs.
- Scissors or Paper Cutter: For cutting your scratch offs to size.
- Design Software: Such as Adobe Photoshop, Illustrator, or free alternatives like GIMP.
- Laser Printer (Optional): For printing on the back of the scratch off material if needed.
Step-by-Step Guide
1. Design Your Scratch Off
- Choose Your Design: Decide on the design you want to appear when the scratch off is revealed. This could be a prize, a message, or an image.
- Create Your Template: Use design software to create a template for your scratch offs. Ensure the design fits the size of your cardstock or paper.
- Add Scratch Off Area: Designate an area on your template where the scratch off coating will be applied. This area should be free of any design elements that you want to remain hidden.
2. Print Your Design
- Print on Cardstock: Print your design onto the cardstock or heavy paper using an inkjet printer. Ensure the print quality is high to avoid any issues with the scratch off coating.
- Optional: Print on Scratch Off Material: If you need to print on the back of the scratch off material, use a laser printer to avoid any issues with the coating.
3. Apply the Scratch Off Coating
- Prepare the Coating: Follow the instructions on the scratch off coating package to prepare the coating solution.
- Apply the Coating: Use a brush, sponge, or airbrush to apply the coating evenly over the designated scratch off area. Allow the coating to dry completely.
- Second Coat (Optional): For a thicker scratch off layer, apply a second coat after the first one has dried.
4. Cut and Finish
- Cut to Size: Once the coating is dry, use scissors or a paper cutter to cut your scratch offs to the desired size.
- Add Finishing Touches: If needed, add any additional elements like stickers, seals, or packaging to enhance the appearance of your scratch offs.
Tips and Tricks
- Test Your Coating: Before applying the coating to all your scratch offs, test it on a small piece of cardstock to ensure the thickness and scratchability are to your liking.
- Use Quality Materials: High-quality cardstock and scratch off coating will result in a more professional and durable final product.
- Consider the Audience: Tailor your scratch off design to your audience. For example, use bright colors and fun graphics for children, and more sophisticated designs for adults.
Applications
Custom scratch offs can be used in a variety of ways:
- Marketing Campaigns: Create promotional scratch offs for product launches or events.
- Casino Nights: Add a fun element to your casino night with custom scratch offs as prizes.
- Personal Projects: Use scratch offs for personalized gifts, party favors, or educational tools.
By following this guide, you can create custom scratch offs that are both fun and functional, tailored to your specific needs and audience.

About create a javascript slot machine FAQ
🤔 How can I create a slot machine game using JavaScript?
Creating a slot machine game in JavaScript involves several steps. First, set up the HTML structure with elements for the reels and buttons. Use CSS to style these elements, ensuring they resemble a traditional slot machine. Next, write JavaScript to handle the game logic. This includes generating random symbols for each reel, spinning the reels, and checking for winning combinations. Implement functions to calculate winnings based on the paylines. Add event listeners to the spin button to trigger the game. Finally, use animations to make the spinning reels look realistic. By following these steps, you can create an engaging and interactive slot machine game using JavaScript.
🤔 How can I create a slot machine using HTML and JavaScript?
Creating a slot machine using HTML and JavaScript involves several steps. First, design the layout using HTML, including reels and buttons. Use CSS for styling, ensuring a visually appealing interface. Next, implement the slot machine logic in JavaScript. Create functions to spin the reels, calculate outcomes, and handle user interactions. Use arrays to represent reel symbols and randomize their positions on each spin. Add event listeners to buttons for starting and stopping the spin. Finally, update the display dynamically based on the results. This approach combines front-end design with interactive functionality, offering a fun and engaging user experience.
🤔 How to Create a JavaScript Slot Machine?
Creating a JavaScript slot machine involves several steps. First, set up the HTML structure with slots and a button. Use CSS for styling, ensuring the slots are aligned. In JavaScript, generate random symbols for each slot. Implement a function to check if the symbols match when the button is clicked. If they match, display a win message; otherwise, show a loss message. Use event listeners to handle button clicks and update the slot symbols dynamically. This project enhances your JS skills and provides an interactive web experience. Remember to test thoroughly for responsiveness and functionality across different devices.
🤔 How can I create a slot machine script for a game or simulation?
Creating a slot machine script involves several steps. First, define the symbols and their probabilities. Next, use a random number generator to simulate spins. Display the results and calculate winnings based on predefined paylines and rules. Implement a loop for continuous play and manage the player's balance. Use programming languages like Python or JavaScript for scripting. Ensure the script handles edge cases and provides feedback to the player. Test thoroughly to ensure fairness and accuracy. This approach will help you create an engaging and functional slot machine simulation.
🤔 How to Create a Slot Machine Using HTML5: A Step-by-Step Tutorial?
Creating a slot machine using HTML5 involves several steps. First, design the layout with HTML, including reels and buttons. Use CSS for styling, ensuring a visually appealing interface. Next, implement JavaScript to handle the slot machine's logic, such as spinning the reels and determining outcomes. Use event listeners to trigger spins and update the display. Finally, test thoroughly for responsiveness and functionality across different devices. This tutorial provides a foundational understanding, enabling you to create an interactive and engaging slot machine game.
🤔 How to Create a JavaScript Slot Machine?
Creating a JavaScript slot machine involves several steps. First, set up the HTML structure with slots and a button. Use CSS for styling, ensuring the slots are aligned. In JavaScript, generate random symbols for each slot. Implement a function to check if the symbols match when the button is clicked. If they match, display a win message; otherwise, show a loss message. Use event listeners to handle button clicks and update the slot symbols dynamically. This project enhances your JS skills and provides an interactive web experience. Remember to test thoroughly for responsiveness and functionality across different devices.
🤔 How can I create a slot machine script for a game or simulation?
Creating a slot machine script involves several steps. First, define the symbols and their probabilities. Next, use a random number generator to simulate spins. Display the results and calculate winnings based on predefined paylines and rules. Implement a loop for continuous play and manage the player's balance. Use programming languages like Python or JavaScript for scripting. Ensure the script handles edge cases and provides feedback to the player. Test thoroughly to ensure fairness and accuracy. This approach will help you create an engaging and functional slot machine simulation.
🤔 What Steps Are Needed to Build a JavaScript Slot Machine?
To build a JavaScript slot machine, start by creating the HTML structure with slots and buttons. Use CSS for styling, ensuring a visually appealing layout. Implement JavaScript to handle the logic: generate random symbols, spin the slots, and check for winning combinations. Attach event listeners to the spin button to trigger the animation and result checking. Ensure the game handles wins and losses gracefully, updating the display accordingly. Test thoroughly for bugs and responsiveness across devices. By following these steps, you'll create an engaging and functional JavaScript slot machine.
🤔 How to Create a Slot Machine Using HTML5: A Step-by-Step Tutorial?
Creating a slot machine using HTML5 involves several steps. First, design the layout with HTML, including reels and buttons. Use CSS for styling, ensuring a visually appealing interface. Next, implement JavaScript to handle the slot machine's logic, such as spinning the reels and determining outcomes. Use event listeners to trigger spins and update the display. Finally, test thoroughly for responsiveness and functionality across different devices. This tutorial provides a foundational understanding, enabling you to create an interactive and engaging slot machine game.
🤔 How to Create a JavaScript Slot Machine?
Creating a JavaScript slot machine involves several steps. First, set up the HTML structure with slots and a button. Use CSS for styling, ensuring the slots are aligned. In JavaScript, generate random symbols for each slot. Implement a function to check if the symbols match when the button is clicked. If they match, display a win message; otherwise, show a loss message. Use event listeners to handle button clicks and update the slot symbols dynamically. This project enhances your JS skills and provides an interactive web experience. Remember to test thoroughly for responsiveness and functionality across different devices.